Table of Contents
ToggleStored Procedures in SQL Server
Stored Procedures in SQL Server serve as precompiled collections of SQL statements that can be executed as a single unit, providing a powerful way to encapsulate business logic within the database. The primary benefits of Stored Procedures include improved performance due to precompilation and caching of execution plans, enhanced security by controlling access and reducing the risk of SQL injection, and greater code reusability and maintainability by centralizing logic that can be consistently applied across multiple applications. Additionally, Stored Procedures support parameterization, making them flexible and efficient for handling complex operations, and they facilitate better error handling and transaction management within the database.
Use Stored Procedures for Reusable Logic
Encapsulation: Use Stored Procedures to encapsulate frequently used logic or business rules, ensuring application consistency and simplifying maintenance.
Modularity: Break complex logic into smaller, modular Stored Procedures that can be reused in different parts of your application.
Parameterization
Input Parameters: Use input parameters to pass data into your Stored Procedures, making them flexible and reusable.
Output Parameters: Use output parameters or return values to return data from Stored Procedures when necessary.
Error Handling
TRY…CATCH Blocks: Implement error handling within Stored Procedures using TRY...CATCH
blocks to manage exceptions and ensure graceful failure.
Logging: Consider logging errors within the CATCH
block to a dedicated error table or using an external logging mechanism.
Performance Optimization
Indexes: Ensure that the tables referenced in your Stored Procedures are properly indexed to improve performance.
Execution Plan Review: Regularly review execution plans using SQL Server’s built-in tools to identify and optimize slow queries within your Stored Procedures.
Avoid Cursors: Where possible, avoid using cursors as they can be slow and resource-intensive. Use set-based operations instead.
Security
Permissions: Restrict access to Stored Procedures by granting execute permissions only to specific roles or users.
SQL Injection Prevention: Protect against SQL injection by using parameterized queries within your Stored Procedures and avoiding dynamic SQL wherever possible.
Documentation and Naming Conventions
Clear Naming: Use clear and consistent naming conventions for your Stored Procedures to make their purpose easily identifiable. For example, prefix with usp_
(user Stored Procedure) or sp_
(system Stored Procedure).
Documentation: Document the purpose, parameters, and expected behavior of Stored Procedures within the code using comments. This aids in understanding and maintaining the code over time.
Version Control
Source Control: Store your Stored Procedures in a source control system like Git. This allows for tracking changes, versioning, and collaboration.
Change Management: Implement a change management process for deploying Stored Procedure updates to ensure that changes are properly tested and documented.
Testing
Unit Testing: Write unit tests for your Stored Procedures to ensure they behave as expected under various conditions.
Performance Testing: Test the performance of Stored Procedures under load to ensure they can handle production workloads efficiently.
Avoid Over-Complexity
Single Responsibility: Ensure each Stored Procedure is responsible for a single task or operation. Avoid creating Stored Procedures that try to do too much or handle too many different scenarios.
Refactoring: Periodically review and refactor Stored Procedures to simplify logic, improve performance, or incorporate new SQL Server features.
Monitoring and Maintenance
Regular Review: Periodically review Stored Procedures for performance issues, deprecated features, or opportunities for optimization.
Cleanup: Remove or archive unused Stored Procedures to keep the database schema clean and maintainable.
Example: Stored Procedure for Retrieving Sales Data
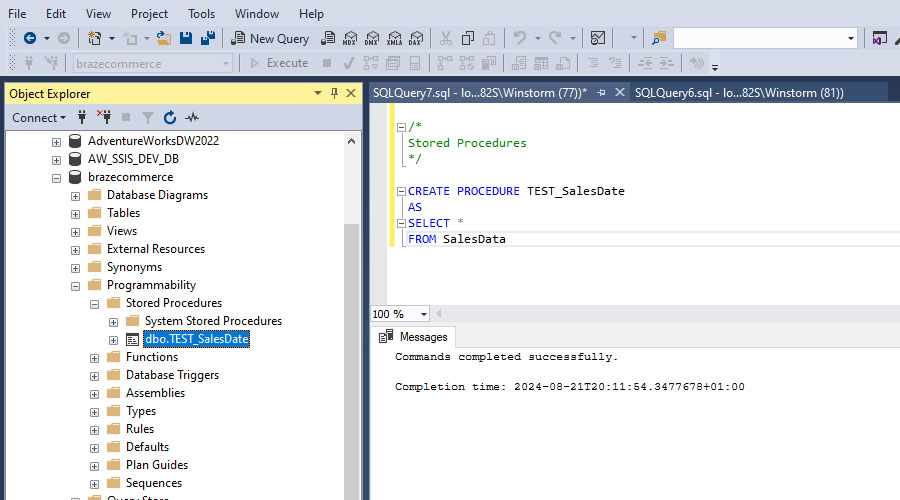
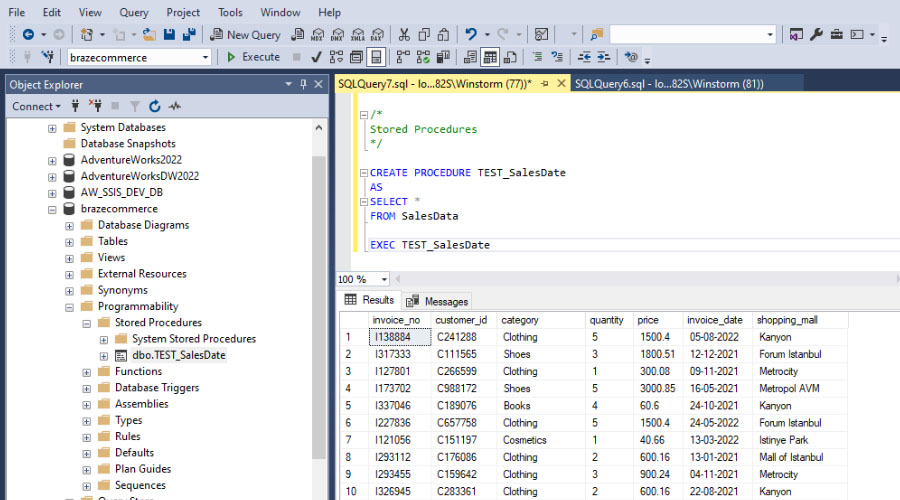
Example: Calculating Total Sales for a Product
SQL Query / command:
create procedure
*/
CREATE PROCEDURE GetTotalSalesByProduct
@ProductID INT,
@StartDate DATE,
@EndDate DATE
AS
BEGIN
— Set NOCOUNT ON to prevent extra result sets from interfering with SELECT statements.
SET NOCOUNT ON;
— Calculate the total sales amount for the specified product within the date range
SELECT
@ProductID AS ProductID,
SUM(SaleAmount) AS TotalSalesAmount,
SUM(Quantity) AS TotalQuantitySold
FROM
Sales
WHERE
ProductID = @ProductID
AND SaleDate BETWEEN @StartDate AND @EndDate;
END;
———————————————————————————————————————————————–
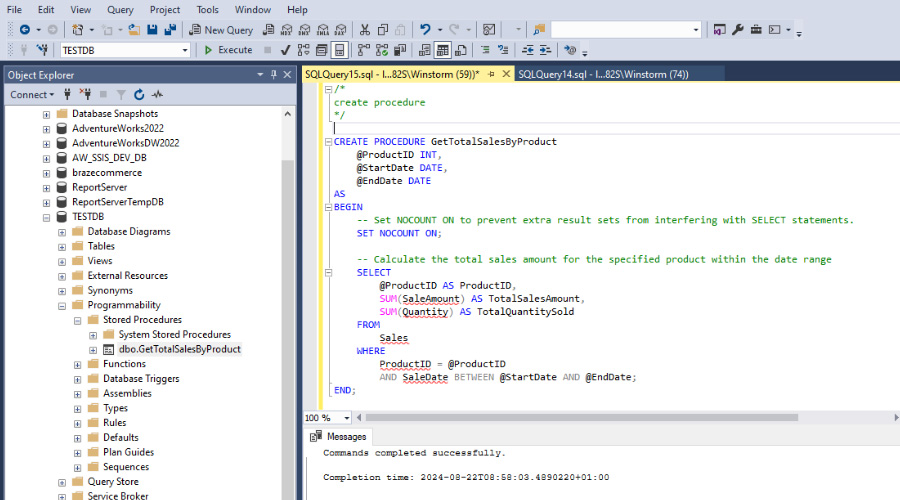
To execute this Stored Procedure and calculate the total sales for ProductID = 101 between 2023-08-01 and 2023-12-31, you would run the following command:
EXEC GetTotalSalesByProduct @ProductID = 101, @StartDate = ‘2023-08-01’, @EndDate = ‘2023-12-31’;
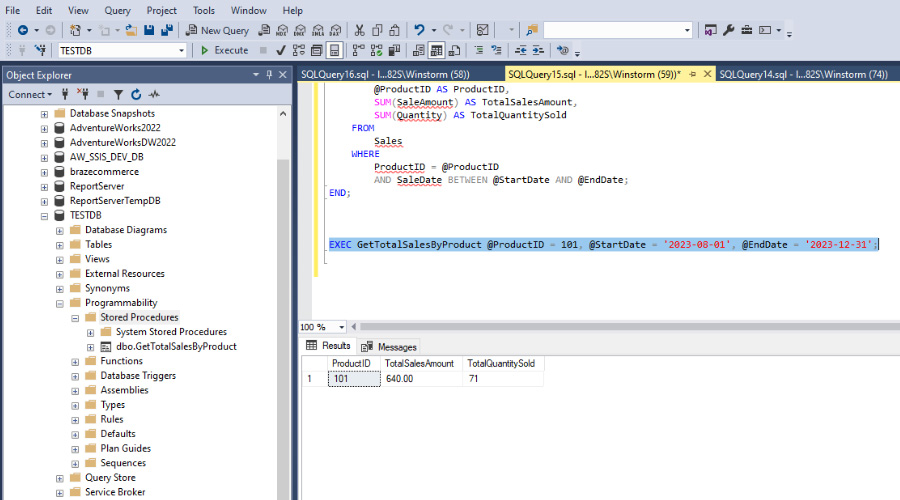
This Stored Procedure efficiently calculates the total sales and quantity sold for a specific product within a specified date range, making it a useful tool for sales reporting and analysis.
Example: Retrieving Employees by Department and Salary
SQL Query / Command:
/*
create procedure
*/
CREATE PROCEDURE GetEmployeesByDepartmentAndSalary
@Department NVARCHAR(50),
@SalaryThreshold DECIMAL(18, 2)
AS
BEGIN
— Set NOCOUNT ON to prevent extra result sets from interfering with SELECT statements.
SET NOCOUNT ON;
— Select statement to retrieve the employees based on department and salary threshold
SELECT
EmployeeID,
FirstName,
LastName,
Department,
Salary
FROM
Employees
WHERE
Department = @Department
AND Salary > @SalaryThreshold
ORDER BY
Salary DESC;
END;
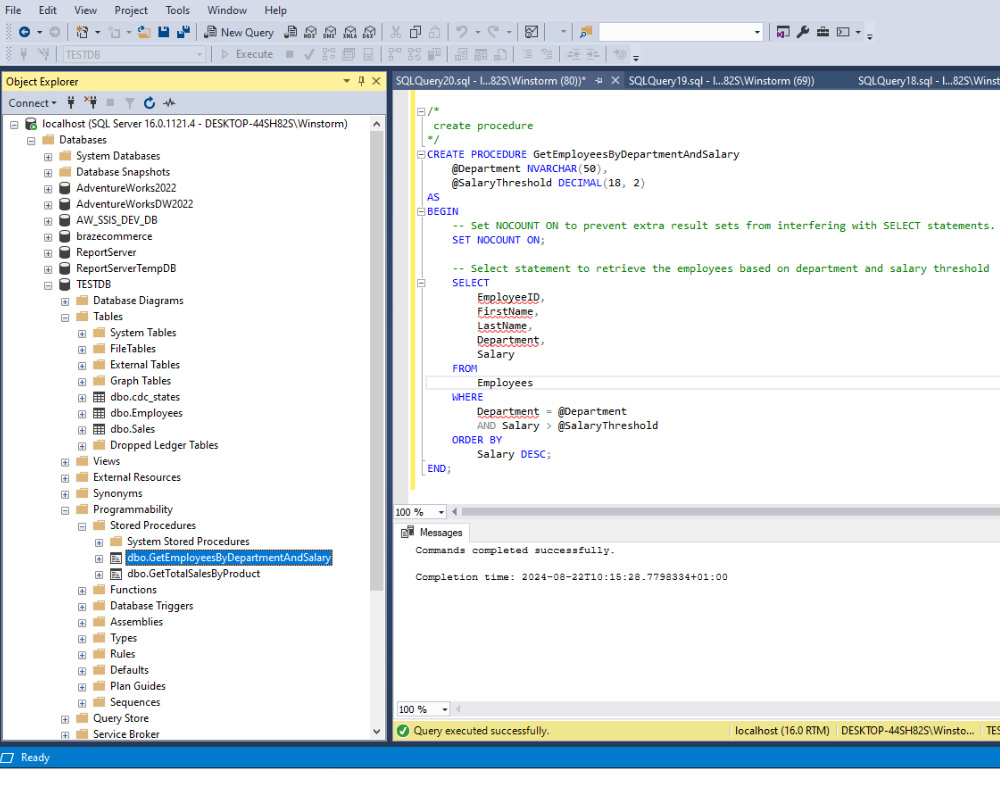
Executing the Stored Procedure
To execute this Stored Procedure and retrieve employees from the “IT” department with a salary greater than 50,000, you would run the following command:
EXEC GetEmployeesByDepartmentAndSalary @Department = ‘IT’, @SalaryThreshold = 50000;
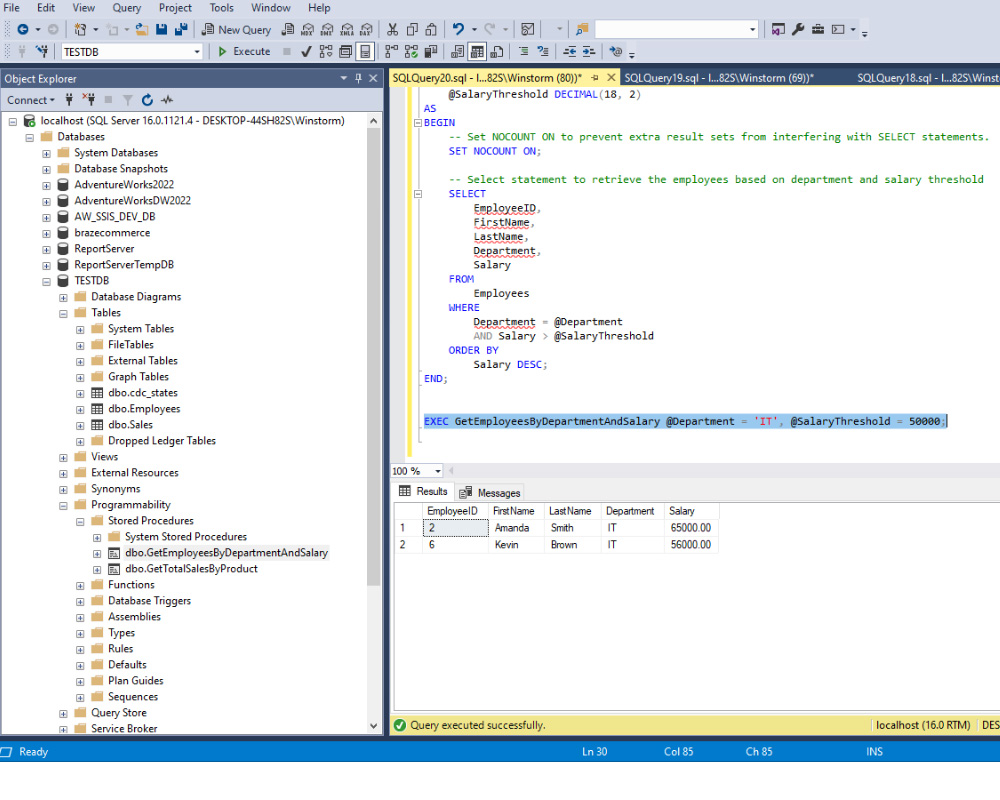
This Stored Procedure can be reused whenever you need to retrieve employees based on department and salary, making it a powerful and reusable piece of database logic.
Trademark Disclaimer:
All trademarks, logos, and brand names are the property of their respective owners. All company, product, and service names used in this website are for identification purposes only. Use of these names trademarks, and brands do not imply endorsement.