Table of Contents
ToggleT-SQL / Transact-SQL
T-SQL, or Transact-SQL, is an extension of SQL (Structured Query Language) developed by Microsoft and Sybase. It is specifically designed to work with Microsoft SQL Server and Sybase ASE (Adaptive Server Enterprise) databases. T-SQL adds several features to the standard SQL language, making it a powerful tool for managing and manipulating relational databases in the Microsoft ecosystem.
Here are some key features and aspects of T-SQL:
Procedural Programming: T-SQL supports procedural programming constructs such as variables, control-of-flow language (IF…ELSE statements, loops), and error handling with TRY…CATCH blocks. This allows for more complex logic and automation within SQL scripts and stored procedures.
System Functions: T-SQL provides a wide range of built-in functions for string manipulation, date and time operations, mathematical calculations, and more. These functions enhance the capabilities of SQL queries and procedures.
System Procedures: T-SQL allows the execution of system stored procedures. These are pre-defined procedures provided by the database system for performing specific tasks. For instance, there are system procedures for backup, restore, and database maintenance tasks.
Error Handling: T-SQL introduces structured error handling using the TRY…CATCH blocks. This feature allows developers to handle errors more effectively, providing mechanisms for trapping and handling errors in a controlled manner.
Transaction Control: T-SQL supports explicit transactions, allowing developers to define and control transaction boundaries explicitly. This ensures data integrity and consistency by allowing multiple SQL statements to be treated as a single unit of work.
Enhanced Security: T-SQL includes features like stored procedures and views, which can be used to encapsulate complex logic and restrict access to certain data elements. This enhances security by allowing fine-grained control over database access.
Integration with .NET: T-SQL can be integrated with the .NET framework, allowing developers to write stored procedures, triggers, and functions using .NET languages like C# and Visual Basic.NET. This integration enables the use of the full power of .NET within the database.
Data Manipulation and Reporting: T-SQL supports various data manipulation operations, including SELECT (for querying data), INSERT (for adding new records), UPDATE (for modifying existing records), and DELETE (for removing records). It is also used for creating views, stored procedures, and functions, enabling efficient data reporting and analysis.
The fundamental T-SQL statements
These are just some of the fundamental T-SQL statements. T-SQL also includes various functions, stored procedures, triggers, and more. For detailed information, you may want to refer to the official Microsoft documentation or other authoritative T-SQL resources.
SELECT:
Retrieve data from one or more tables.
SELECT column1, column2 FROM table_name WHERE condition;
INSERT:
Add records to a table.
INSERT INTO table_name (column1, column2) VALUES (value1, value2);
UPDATE:
Modify existing records in a table.
UPDATE table_name SET column1 = value1 WHERE condition;
DELETE:
Remove records from a table.
DELETE FROM table_name WHERE condition;
CREATE TABLE:
Create a new table with specified columns and data types.
CREATE TABLE table_name (
column1 datatype,
column2 datatype
);
ALTER TABLE:
Modify an existing table (add, modify, or drop columns).
ALTER TABLE table_name
ADD column_name datatype;
ALTER TABLE table_name
MODIFY column_name datatype;
ALTER TABLE table_name
DROP COLUMN column_name;
CREATE INDEX:
Create an index on one or more columns to improve query performance.
CREATE INDEX index_name
ON table_name (column1, column2);
DROP TABLE:
Delete an existing table and its data.
DROP TABLE table_name;
WHERE Clause:
Filter records based on a specified condition.
SELECT column1, column2 FROM table_name WHERE condition;
GROUP BY:
Group rows that have the same values in specified columns.
SELECT column1, COUNT(*)
FROM table_name
GROUP BY column1;
HAVING Clause:
Filter groups based on a condition.
SELECT column1, COUNT(*)
FROM table_name
GROUP BY column1
HAVING COUNT(*) > 1;
ORDER BY:
Sort the result set based on one or more columns.
SELECT column1, column2
FROM table_name
ORDER BY column1 ASC, column2 DESC;
DISTINCT:
The DISTINCT
keyword is used in the SELECT
statement to retrieve unique rows from a result set. Here’s an example:
SELECT DISTINCT column1, column2
FROM table_name;
JOIN:
Combine rows from two or more tables based on a related column.
SELECT *
FROM table1
INNER JOIN table2 ON table1.column = table2.column;
INNER JOIN, LEFT JOIN, RIGHT JOIN:
Different types of joins to control the inclusion of non-matching rows.
SELECT *
FROM table1
INNER JOIN table2 ON table1.column = table2.column;
— LEFT JOIN
SELECT *
FROM table1
LEFT JOIN table2 ON table1.column = table2.column;
— RIGHT JOIN
SELECT *
FROM table1
RIGHT JOIN table2 ON table1.column = table2.column;
UNION:
Combine the result sets of two or more SELECT statements.
SELECT column1 FROM table1
UNION
SELECT column1 FROM table2;
SUBQUERIES:
Nest a SELECT statement within another statement.
SELECT column1
FROM table1
WHERE column2 IN (SELECT column2 FROM table2);
CASE Statement:
Perform conditional logic within a query.
SELECT
column1,
CASE
WHEN column2 > 10 THEN ‘High’
WHEN column2 > 5 THEN ‘Medium’
ELSE ‘Low’
END AS Priority
FROM table1;
GROUP_CONCAT (STRING_AGG in SQL Server 2017 and later):
Concatenate values from multiple rows into a single string.
SELECT
column1,
STRING_AGG(column2, ‘, ‘) AS ConcatenatedValues
FROM table1
GROUP BY column1;
Stored Procedures:
Stored sets of SQL statements for reuse.
CREATE PROCEDURE sp_GetEmployee
AS
BEGIN
SELECT * FROM Employee;
END;
EXEC sp_GetEmployee;
Transactions:
Ensure a series of SQL statements are executed as a single unit.
BEGIN TRANSACTION;
— SQL statements
COMMIT; — or ROLLBACK;
Views:
Virtual tables are based on the result of a SELECT statement.
CREATE VIEW vw_Employees AS
SELECT EmployeeID, FirstName, LastName
FROM Employees
WHERE Department = ‘IT’;
SELECT * FROM vw_Employees;
These are just a few more examples of T-SQL statements and concepts. T-SQL is a powerful language with various features for querying and manipulating data in SQL Server databases. The syntax and availability of certain features may vary depending on the SQL Server version.
CREATE DATABASE
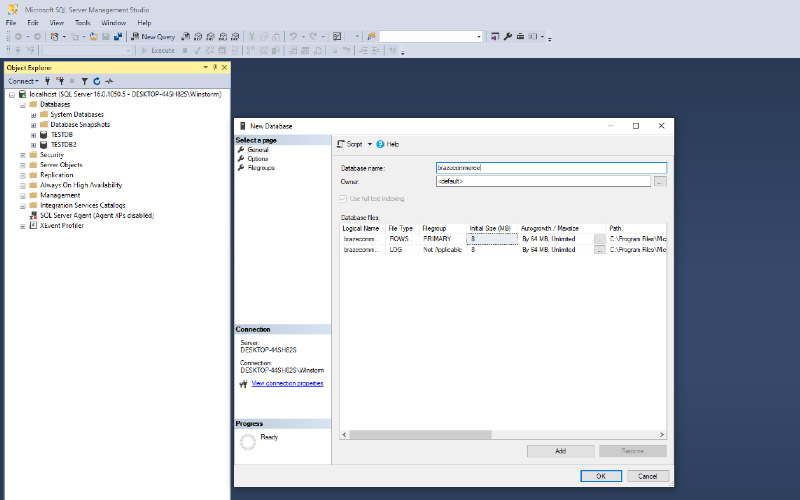
CREATE TABLE
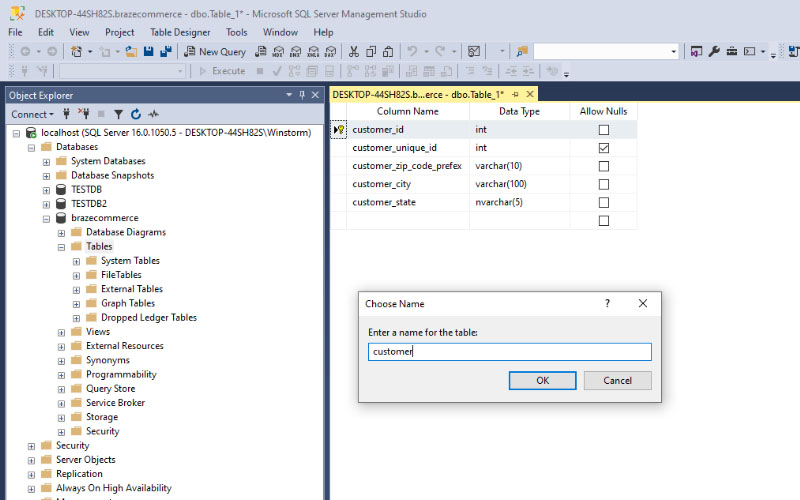
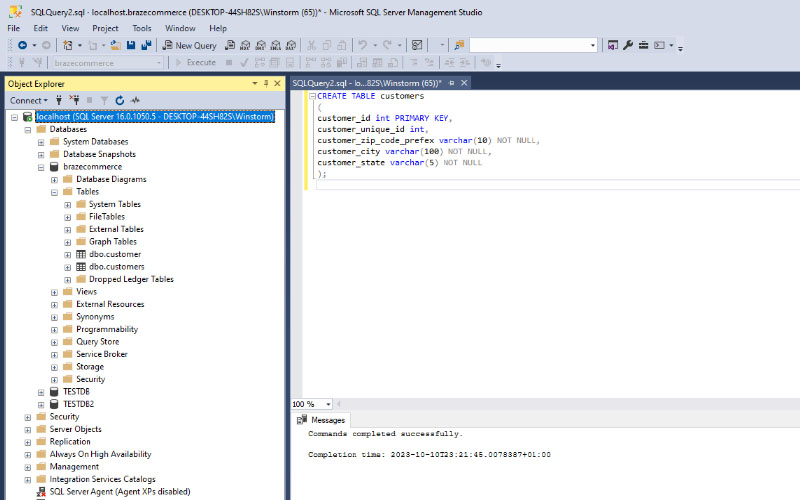
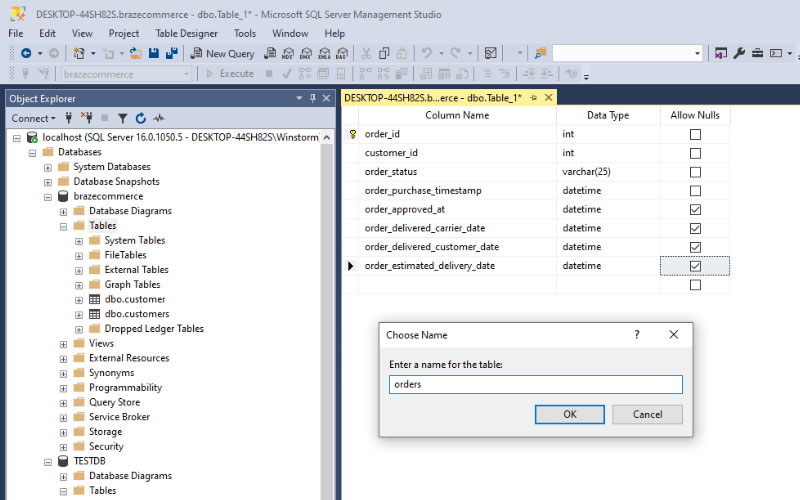
CREATE TABLE with dataset
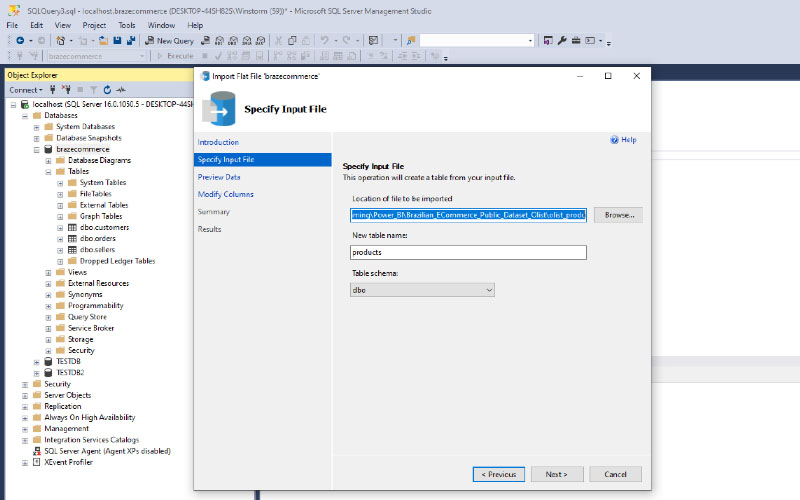
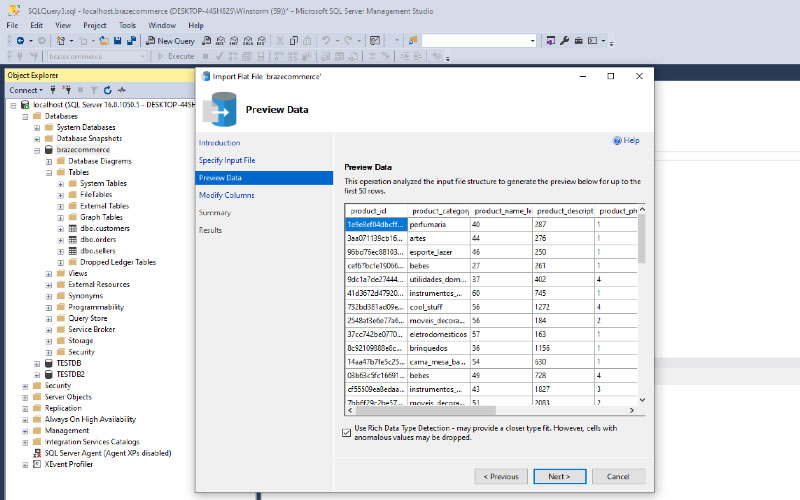
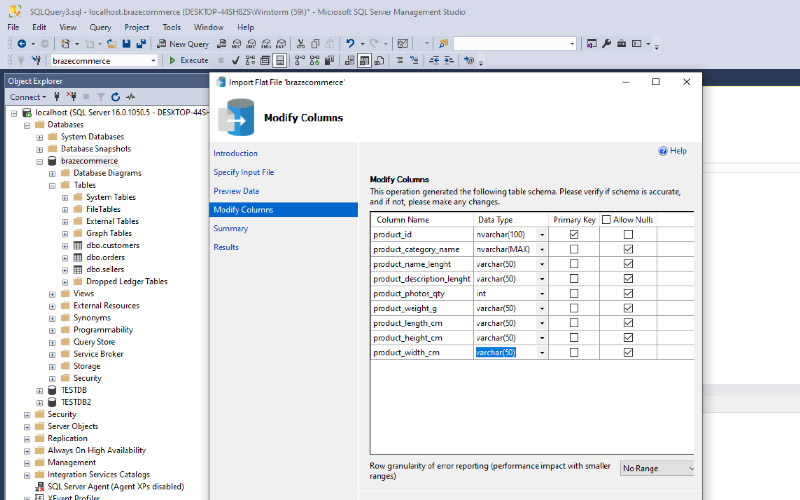
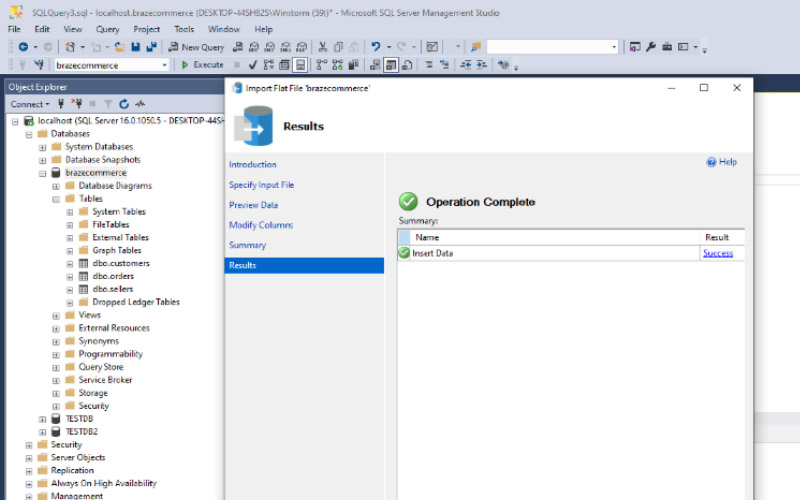
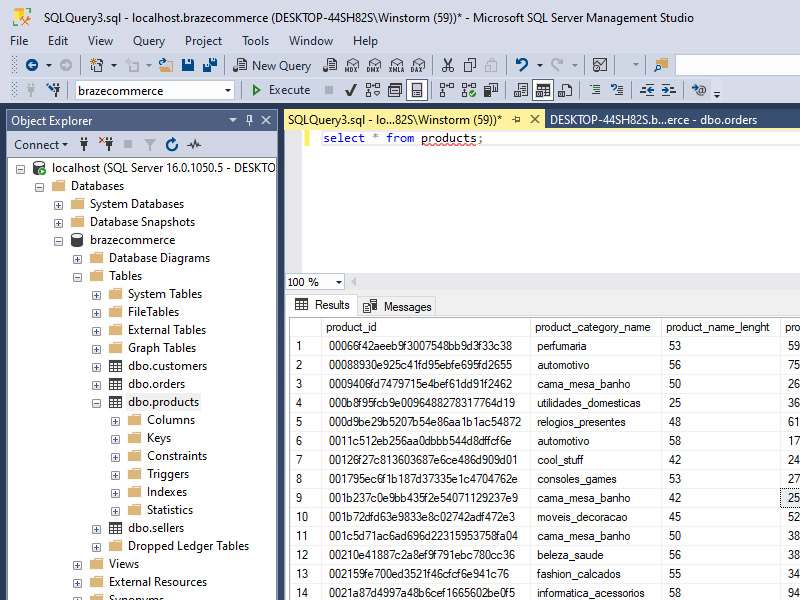
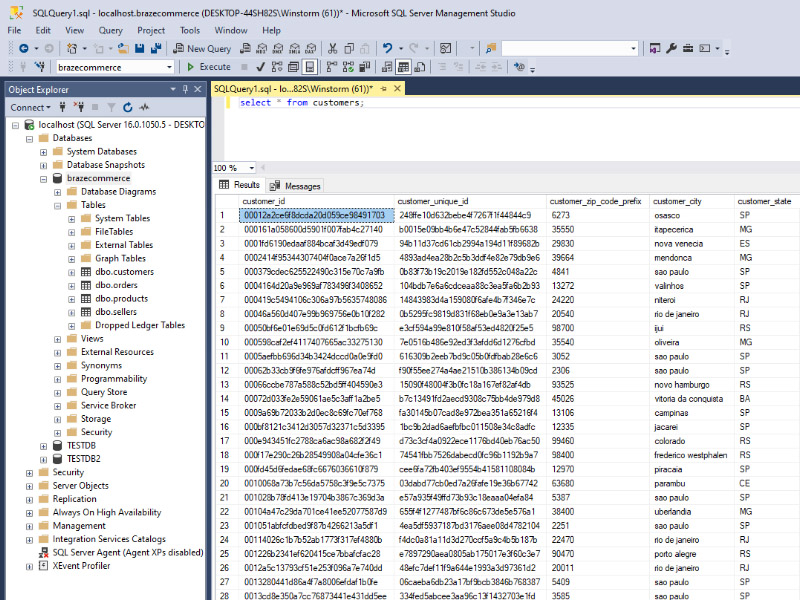
SQL Query
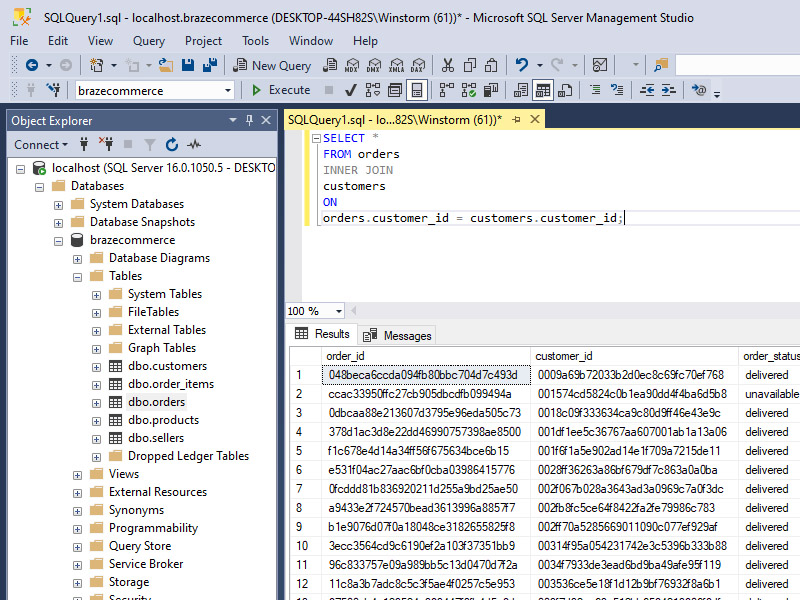
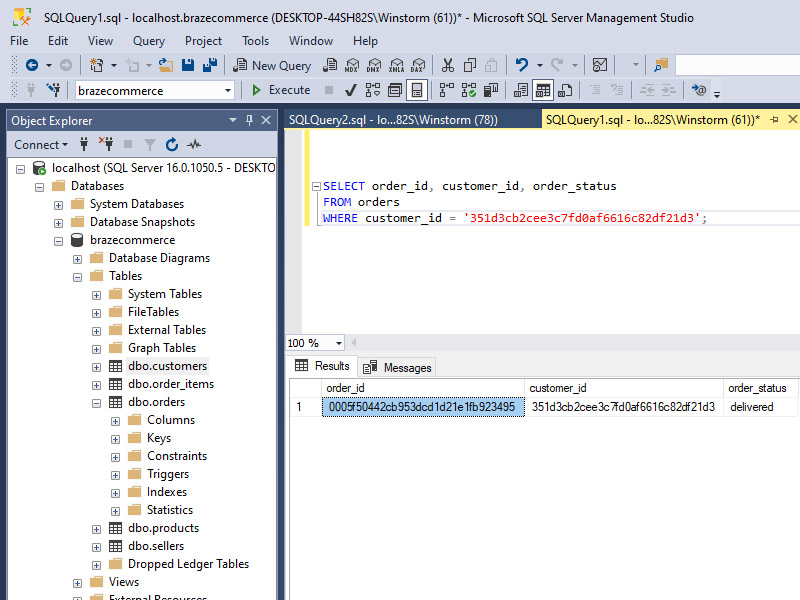
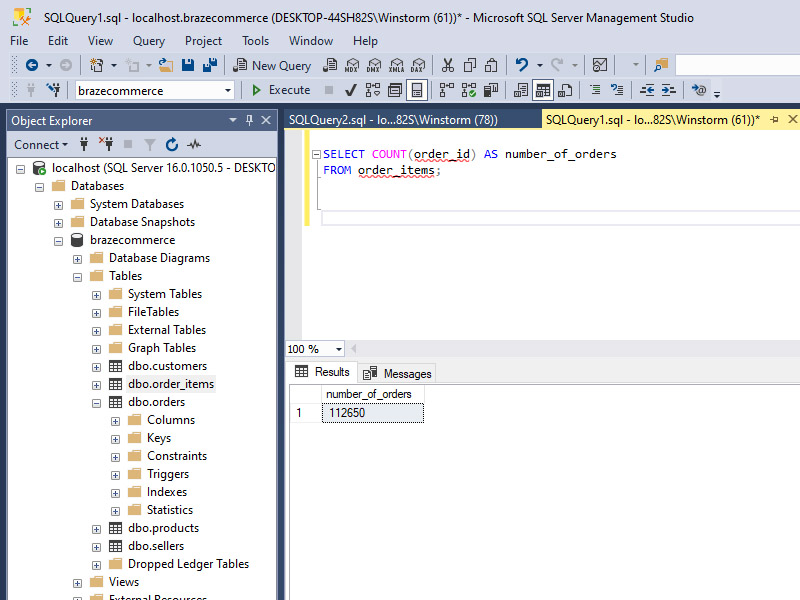
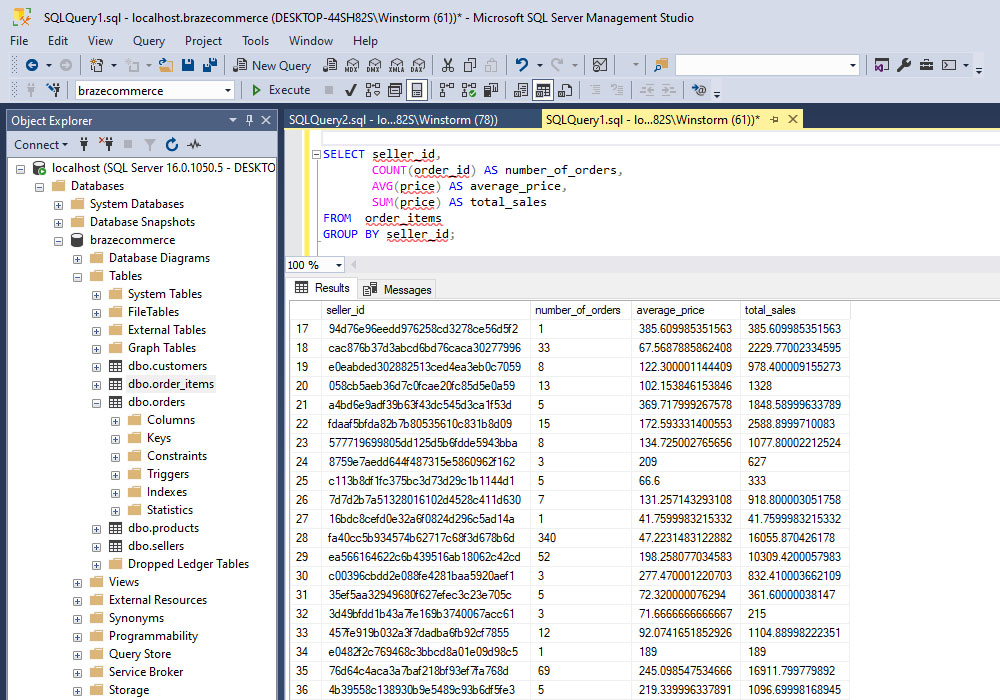
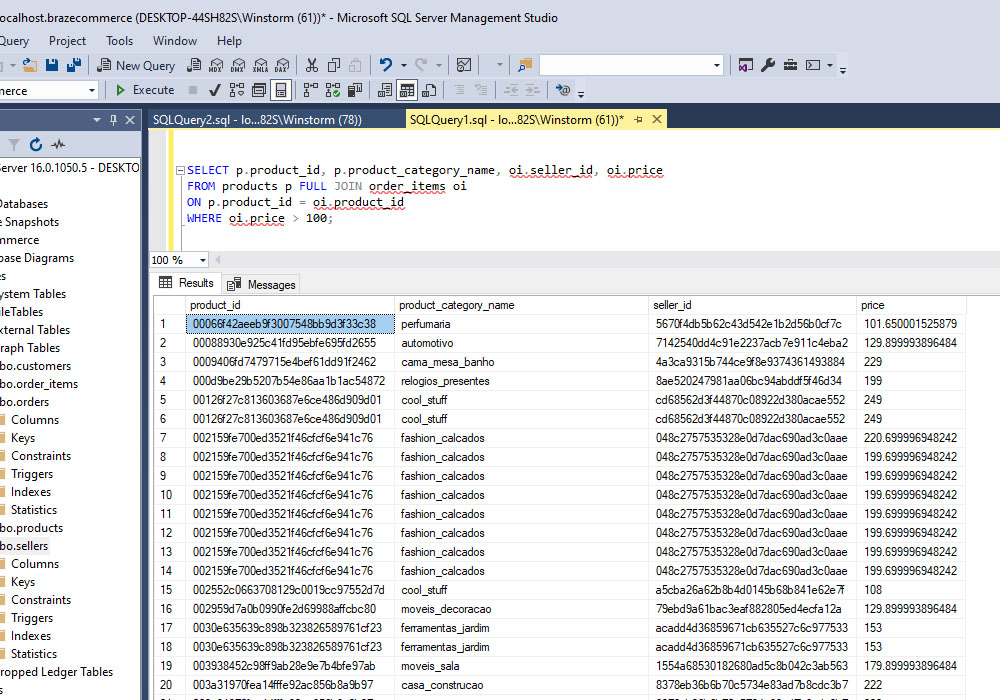
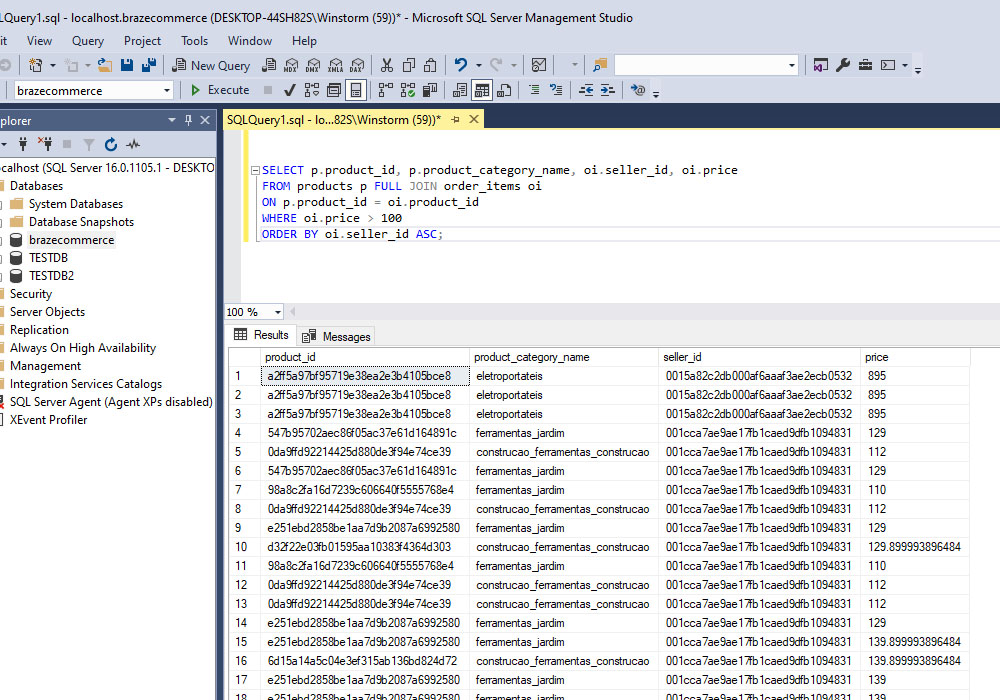
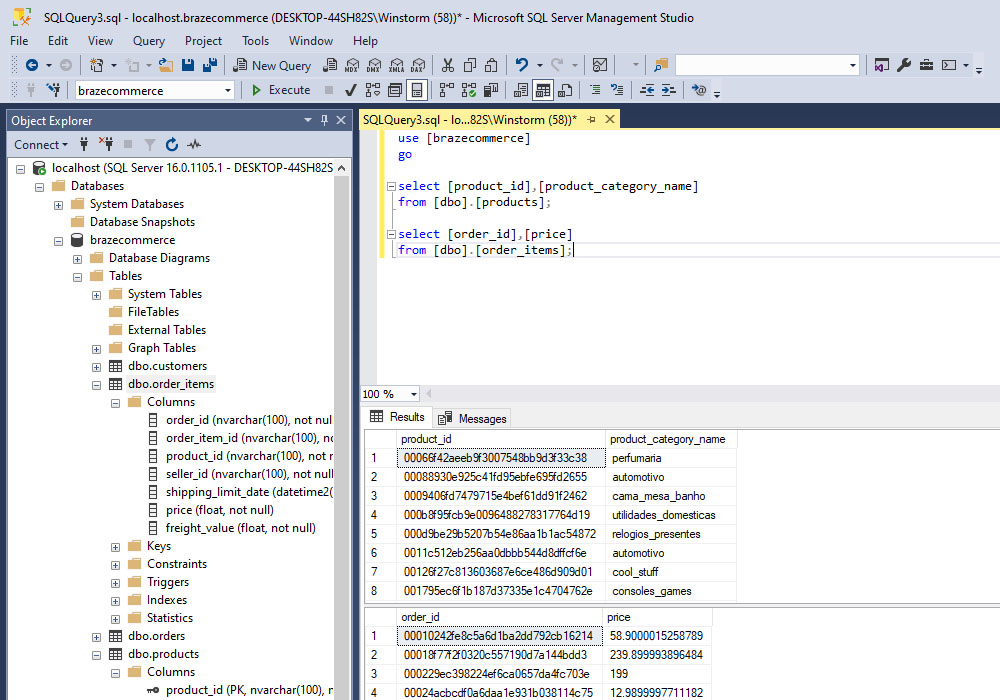
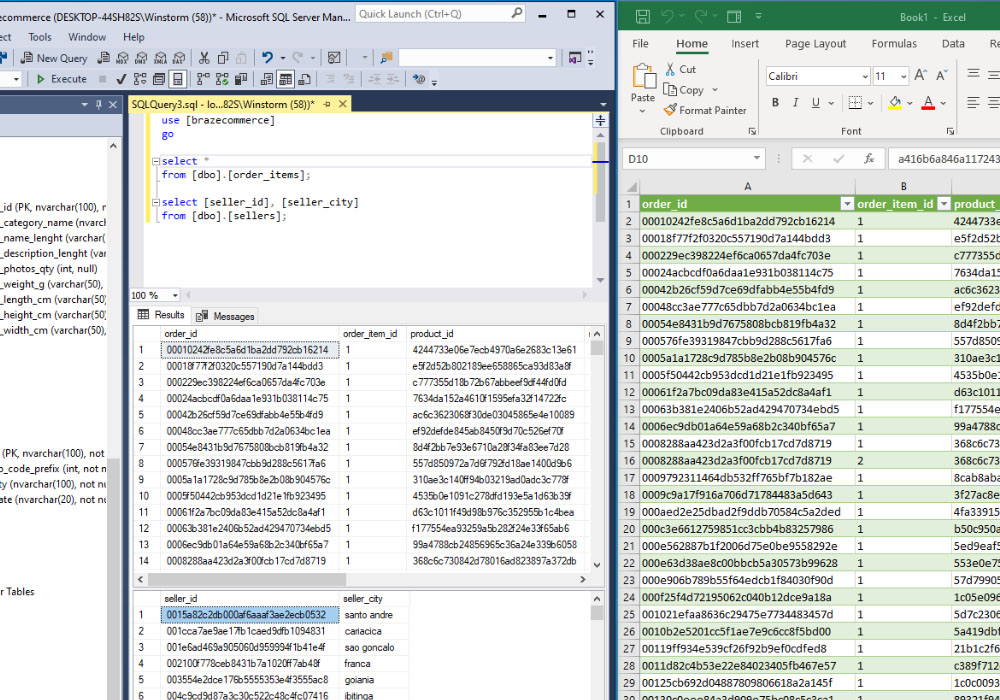
T-SQL is a powerful tool for developers and database administrators working with Microsoft SQL Server and Sybase ASE databases. Its rich feature set and integration capabilities make it a versatile language for managing and manipulating data in the Microsoft database environment.
T-SQL and Power BI
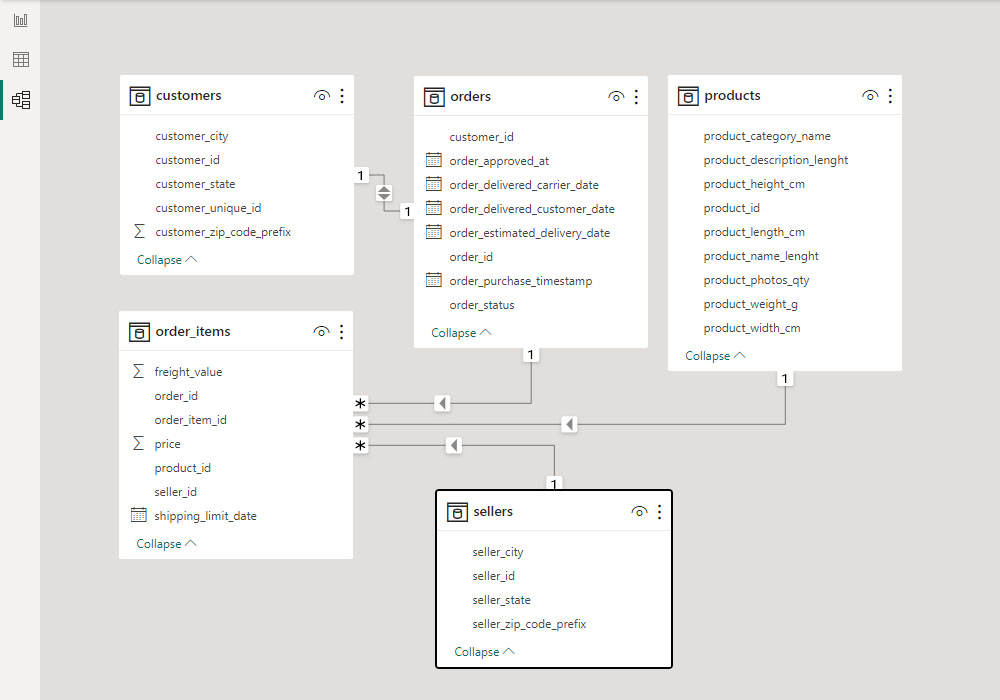
T-SQL (Transact-SQL) and Power BI are two powerful tools in the Microsoft ecosystem that work together seamlessly to help users manage and analyze data. T-SQL is a language used for managing and querying data in Microsoft SQL Server and other related database management systems, while Power BI is a business analytics service provided by Microsoft that allows users to visualize and share insights from their data. Here’s how T-SQL and Power BI are related and how they work together:
1. Data Retrieval and Transformation:
T-SQL: T-SQL is used to retrieve data from SQL Server databases. It can perform complex data manipulation operations, aggregations, filtering, and transformations on the data stored in SQL Server databases.
Power BI: Power BI can connect directly to SQL Server databases using T-SQL queries. Users can write custom T-SQL queries within Power BI to fetch specific data subsets or perform advanced transformations on the data before loading it into the Power BI model.
2. Data Modeling:
T-SQL: T-SQL allows users to create and manage database schemas, tables, relationships, indexes, and other database objects. It also supports the definition of complex views and stored procedures that can be used for data modeling and processing.
Power BI: Power BI has a robust data modeling interface where users can create relationships between different tables imported from various data sources, including SQL Server databases. Power BI automatically detects and creates relationships based on the column names, but users can define custom relationships using T-SQL logic if needed.
3. Data Visualization and Analysis:
T-SQL: While T-SQL is not used for visualization, it can be used to aggregate and process data before it’s presented in Power BI. For example, T-SQL can calculate summary statistics or perform calculations that are then visualized in Power BI.
Power BI: Power BI is primarily used for data visualization and analysis. Users can create interactive reports and dashboards using a drag-and-drop interface. The data retrieved and transformed using T-SQL queries can be visualized in various formats such as charts, graphs, tables, and maps.
4. Data Refresh and Integration:
T-SQL: T-SQL can be used to schedule jobs and stored procedures that update and refresh data in SQL Server databases. These refreshed data sets can be used as a source for Power BI reports.
Power BI: Power BI allows users to schedule automatic data refresh for reports and dashboards. When the underlying data in the SQL Server database changes, Power BI can automatically refresh the data in the reports, ensuring that users always see up-to-date information.
5. Security and Access Control:
T-SQL: T-SQL supports security features such as user roles, permissions, and encryption, allowing administrators to control access to the data stored in SQL Server databases.
Power BI: Power BI integrates with Azure Active Directory and other authentication methods to control access to reports and dashboards. Security roles and permissions can be defined within Power BI, ensuring that users only see the data they are allowed to access.
In summary, T-SQL and Power BI work together to enable seamless data extraction, transformation, modeling, visualization, and analysis processes. T-SQL is used to manage and manipulate the data at the source (SQL Server databases), while Power BI provides a user-friendly interface for creating interactive and visually appealing reports and dashboards based on the processed data. This integration facilitates efficient data-driven decision-making within organizations.